# # =============== dic =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # print(dic) # # # =============== dicread =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # print(dic['boy']) # print(dic['book']) # # # =============== dicget =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # print(dic.get('student')) # print(dic.get('student', '사전에 없는 단어입니다.')) # # # =============== dicin =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # if 'student' in dic: # print("사전에 있는 단어입니다.") # else: # print("이 단어는 사전에 없습니다.") # # # =============== dicchange =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # dic['boy'] = '남자애' # dic['girl'] = '소녀' # del dic['book'] # print(dic) # # # =============== keys =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # print(dic.keys()) # print(dic.values()) # print(dic.items()) # # # =============== keylist =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # keylist = dic.keys() # for key in keylist: # print(key) # # # =============== dicupdate =============== # dic = { 'boy':'소년', 'school':'학교', 'book':'책' } # dic2 = { 'student':'학생', 'teacher':'선생님', 'book':'서적' } # dic.update(dic2) # print(dic) # # # =============== listtodic =============== # li = [ ['boy', '소년'], ['school', '학교'], ['book', '책'] ] # dic = dict(li) # print(dic) # # # =============== alphanum =============== # song = """by the rivers of babylon, there we sat down # yeah we wept, when we remember zion. # when the wicked carried us away in captivity # required from us a song # now how shall we sing the lord's song in a strange land""" # # alphabet = dict() # for c in song: # if c.isalpha() == False: # continue # c = c.lower() # if c not in alphabet: # alphabet[c] = 1 # else: # alphabet[c] += 1 # # print(alphabet) # # # =============== alphanum2 =============== # song = """by the rivers of babylon, there we sat down # yeah we wept, when we remember zion. # when the wicked carried us away in captivity # required from us a song # now how shall we sing the lord's song in a strange land""" # # alphabet = dict() # for c in song: # if c.isalpha() == False: # continue # c = c.lower() # if c not in alphabet: # alphabet[c] = 1 # else: # alphabet[c] += 1 # # key = list(alphabet.keys()) # key.sort() # for c in key: # num = alphabet[c] # print(c, "=>", num) # # # =============== alphanum3 =============== # song = """by the rivers of babylon, there we sat down # yeah we wept, when we remember zion. # when the wicked carried us away in captivity # required from us a song # now how shall we sing the lord's song in a strange land""" # # alphabet = dict() # for c in song: # if c.isalpha() == False: # continue # c = c.lower() # if c not in alphabet: # alphabet[c] = 1 # else: # alphabet[c] += 1 # # for code in range(ord('a'), ord('z') + 1): # c = chr(code) # num = alphabet.get(c, 0) # print(c, "=>", num) # # # =============== set =============== # asia = { 'korea', 'china', 'japan', 'korea' } # print(asia) # # # =============== set2 =============== # print(set("sanghyung")) # print(set([12, 34, 56, 78])) # print(set(("신지희", "한주완", "김태륜"))) # print(set({'boy':'소년', 'school':'학교', 'book':'책'})) # print(set()) # # # =============== setedit =============== # asia = { 'korea', 'china', 'japan' } # asia.add('vietnam') # 추가 # asia.add('china') # 추가 안됨 # asia.remove('japan') # 제거 # print(asia) # # asia.update({'singapore', 'hongkong', 'korea'}) # print(asia) # # # =============== setop =============== # twox = { 2, 4, 6, 8, 10, 12 } # threex = { 3, 6, 9, 12, 15 } # # print("교집합", twox & threex) # print("합집합", twox | threex) # print("차집합", twox - threex) # print("차집합", threex - twox) # print("배타적 차집합", twox ^ threex) # # # =============== setin =============== # mammal = { '코끼리', '고릴라', '사자', '고래', '사람', '원숭이', '개' } # primate = { '사람', '원숭이', '고릴라' } # # if '사자' in mammal: # print("사자는 포유류이다") # else: # print("사자는 포유류가 아니다.") # # print(primate <= mammal) # print(primate < mammal) # print(primate <= primate) # print(primate < primate) # # ########################################################### # ## 11장 ## # # # =============== sequence =============== # score = [ 88, 95, 70, 100, 99 ] # for s in score: # print("성적 :", s) # # # =============== sequence2 =============== # score = [ 88, 95, 70, 100, 99 ] # no = 1 # for s in score: # print(str(no) + "번 학생의 성적 :", s) # no += 1 # # # =============== sequence3 =============== # score = [ 88, 95, 70, 100, 99 ] # for no in range(len(score)): # print(str(no + 1) + "번 학생의 성적 :", score[no]) # # # =============== enumerate =============== # score = [ 88, 95, 70, 100, 99 ] # for no, s in enumerate(score, 1): # print(str(no) + "번 학생의 성적 :", s) # # # =============== zip =============== # yoil = ["월", "화", "수", "목","금", "토", "일"] # food = ["갈비탕", "순대국", "칼국수", "삼겹살"] # menu = zip(yoil, food) # for y, f in menu: # print("%s요일 메뉴 : %s" % (y, f)) # # # =============== anyall =============== # adult = [True, False, True, False ] # print(any(adult)) # print(all(adult)) # # # =============== filter =============== # def flunk(s): # return s < 60 # # score = [ 45, 89, 72, 53, 94 ] # for s in filter(flunk, score): # print(s) # # # =============== map =============== # def half(s): # return s / 2 # # score = [ 45, 89, 72, 53, 94 ] # for s in map(half, score): # print(s, end = ', ') # # # =============== map2 =============== # def total(s, b): # return s + b # # score = [ 45, 89, 72, 53, 94 ] # bonus = [ 2, 3, 0, 0, 5 ] # for s in map(total, score, bonus): # print(s, end=", ") # # # =============== lambda =============== # score = [ 45, 89, 72, 53, 94 ] # for s in filter(lambda x:x < 60, score): # print(s) # # # =============== lambda2 =============== # score = [ 45, 89, 72, 53, 94 ] # for s in map(lambda x:x / 2, score): # print(s, end=", ") # # # =============== varcopy =============== # a = 3 # b = a # print("a = %d, b = %d" % (a, b)) # # a = 5 # print("a = %d, b = %d" % (a, b)) # # # =============== listcopy =============== # list1 = [ 1, 2, 3 ] # list2 = list1 # # list2[1] = 100 # print(list1) # print(list2) # # # =============== listcopy2 =============== # list1 = [ 1, 2, 3 ] # list2 = list1.copy() # # list2[1] = 100 # print(list1) # print(list2) # # # =============== deepcopy =============== # list0 = [ 'a', 'b' ] # list1 = [ list0, 1, 2 ] # list2 = list1.copy() # # list2[0][1] = 'c' # print(list1) # print(list2) # # # =============== deepcopy2 =============== # import copy # # list0 = [ "a", "b" ] # list1 = [ list0, 1, 2 ] # list2 = copy.deepcopy(list1) # # list2[0][1] = "c" # print(list1) # print(list2) # # # =============== is =============== # list1 = [ 1, 2, 3 ] # list2 = list1 # list3 = list1.copy() # # print("1 == 2" , list1 is list2) # print("1 == 3" , list1 is list3) # print("2 == 3" , list2 is list3) # # # =============== varis =============== # a = 1 # b = a # print("a =", a, " b =", b, ":", a is b) # b = 2 # print("a =", a, " b =", b, ":", a is b)
def calscore(name, *score, **option): print(name) sum = 0 for s in score: sum += s print(sum) if (option['avg'] == True ): print("평균 :", sum / len(score)) calscore("홍길동", 88, 99, 77, avg = True) calscore("김한국", 99, 88, 77, avg = False) # 일반 인수인 name은 반드시 첫 번째 인수로 전달. 다음 가변 위치 인수 score가 오는데 점수의 개수는 몇 개든 상관없다. # 함수 호출문의 이름이 없는 인수가 튜플로 묶여 score 인수로 전달된다. # option 가변 키워드 인수에는 성적을 처리하는 방식을 지정하는 옵션 값이 온다.
14 ~26일 까지 Python 수업
PyPI 란?
파이썬의 기본 내장 라이브러리 ( 빌트인 모듈 )의 기능이 한정적이기 때문에 확장 기능을 사용하기 위한 서드파티 라이브러리
---------- 1주차 -------------------
TUE1~4장 변수 타입 연산자
WEN 5~6장 조건문 반복문 함수
THU 8~10장 문자열관리, 리스트와튜플
FRI SQL, html, css selector
다음주 사용 open source
pandas: table data
requests: http client
beautifulsoup: parser for html, xml data. simple than rex
matplotlib: visualization
seaborn: extended visualization based on matplotlib
pymysql: mysql db connector
sqlalchemy: ORM function
*ORM (Object Relational Mapping)
------------ 2주차 ------------------
월 11~15장 컬렉션, 표준모듈, 예외처리, 파일, 클래스
화 16~17장 모듈, 패키지 고급문법
함수에서 키워드 가변 인수
예를들어
큐큐명가에서 자장면을 밥으로 먹었다.
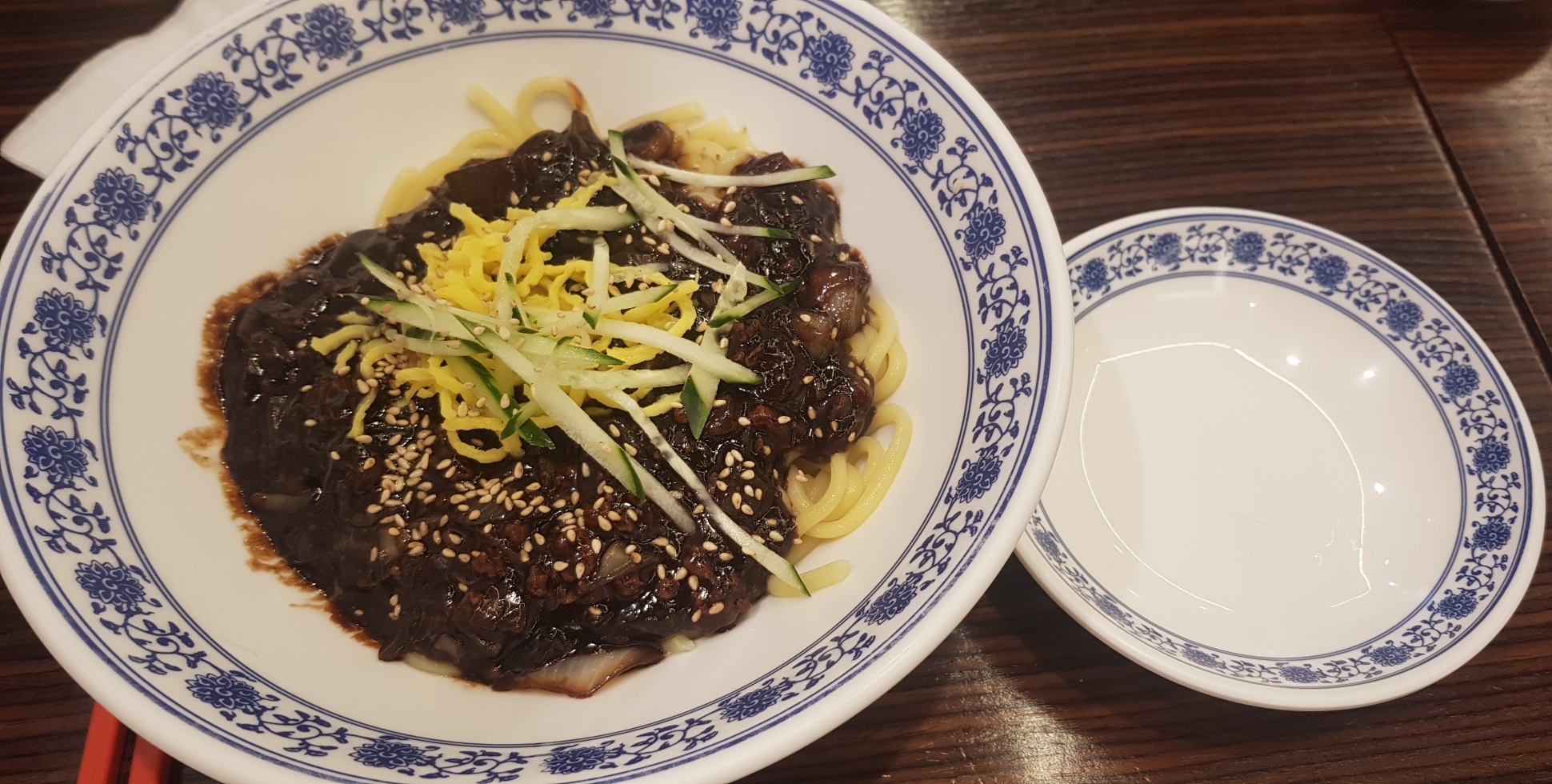
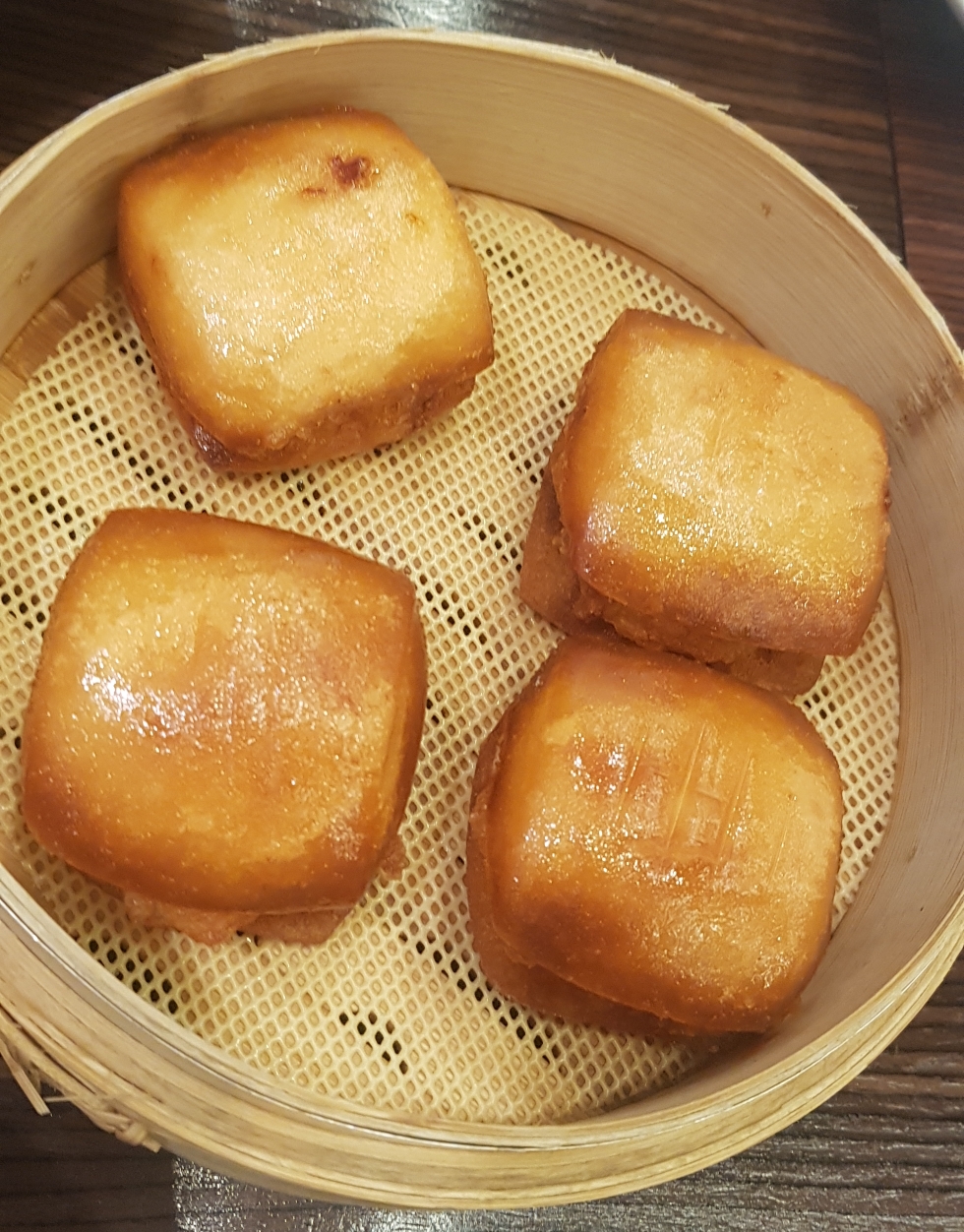